Letter Combinations of a Phone Number - LeetCode
문제요약
2-9의 숫자로 이루어진 문자열이 주어지면, 해당 숫자들로 표현가능한 가능한 문자 조합을 모두 반환
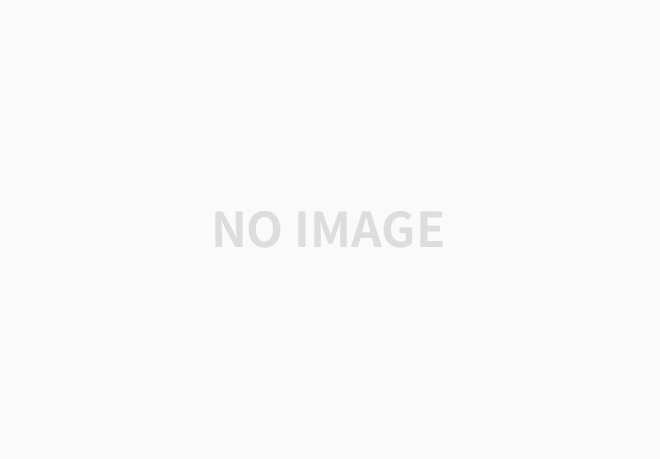
입력
0 <= digits.length <= 4
digits[i] is a digit in the range ['2', '9'].
출력
return string[]
(in any order)
어떻게 풀지?
- 데이터 전처리 : dict로 각 숫자별 사용가능한 char 정리
- 파이썬의 product 라이브러리를 사용하자
- 이 때, map문법과 파이썬의 스프레드 문법(*)을 사용
느낀 점
이번 문제는 문법과 라이브러리만 잘 알면 바로 풀 수 있는 문제였다.
특히, 이번 문제에서 product안에 숫자별 가능한 알파벳 리스트를 담아줄 때, JavaScript의 map과 스프레드 문법이 생각났다.
파이썬에서 map 문법이 있는 건 알았지만, 스프레드 문법은 그간 쓸 일이 없어서 써본 적이 없었고 있는지도 몰랐는데 역시나 있었다.
하나의 언어에 기본적으로 있는 기능은 다른 언어에도 기본적으로 있을 확률이 높은 것 같다.(특히 나중에 나왔을수록…파이썬은 아마 거의 다 있을듯)
다른 언어도 알면 이런 점이 좋구나 생각했다.
- python map
https://www.geeksforgeeks.org/python-map-function/
- python spread operator
https://how.wtf/spread-operator-in-python.html
내 코드
from itertools import product
digits = '23'
def solution(digits):
if digits == '':
return []
dict = {}
dict['2'] = ['a', 'b', 'c']
dict['3'] = ['d', 'e', 'f']
dict['4'] = ['g', 'h', 'i']
dict['5'] = ['j', 'k', 'l']
dict['6'] = ['m', 'n', 'o']
dict['7'] = ['p', 'q', 'r', 's']
dict['8'] = ['t', 'u', 'v']
dict['9'] = ['w', 'x', 'y', 'z']
def find_strs(int_str):
return dict[int_str]
print(list(digits))
print(list(map(find_strs, list(digits))))
res = list(product(*list(map(find_strs, list(digits))), repeat=1))
answer = [''.join(x) for x in res]
return answer
print(solution(digits))
'알고리즘 문제(Python) > LeetCode' 카테고리의 다른 글
[LeetCode/Medium/TwoPointers] Valid Triangle Number(Python) (2) | 2022.11.10 |
---|